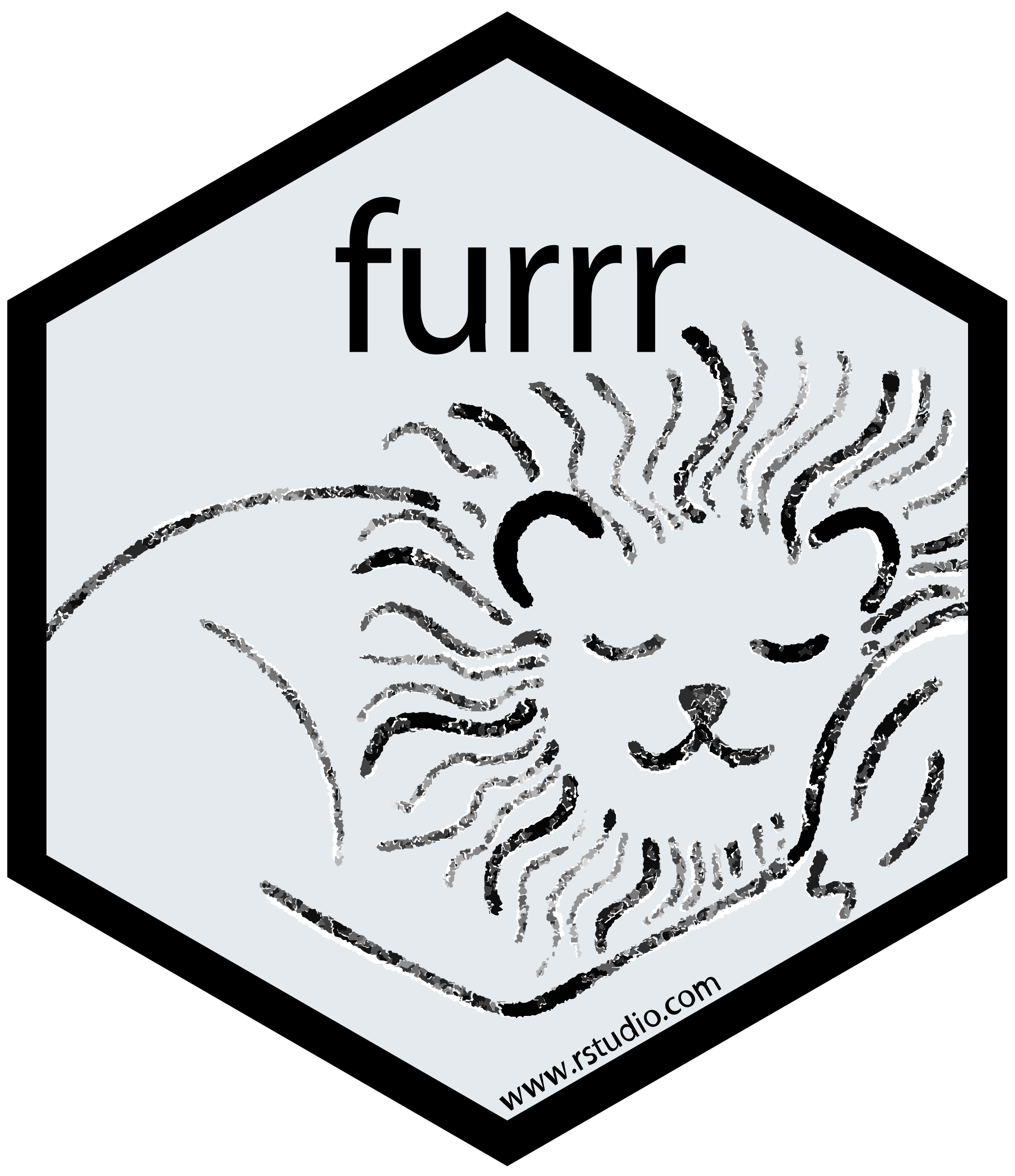
Apply a function to each element of a vector, and its index via futures
Source:R/future-imap.R
, R/future-walk.R
future_imap.Rd
These functions work exactly the same as purrr::imap()
functions,
but allow you to map in parallel.
Usage
future_imap(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_imap_chr(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_imap_dbl(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_imap_int(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_imap_lgl(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_imap_raw(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_imap_dfr(
.x,
.f,
...,
.id = NULL,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_imap_dfc(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
future_iwalk(
.x,
.f,
...,
.options = furrr_options(),
.env_globals = parent.frame(),
.progress = FALSE
)
Arguments
- .x
A list or atomic vector.
- .f
A function, formula, or vector (not necessarily atomic).
If a function, it is used as is.
If a formula, e.g.
~ .x + 2
, it is converted to a function. There are three ways to refer to the arguments:For a single argument function, use
.
For a two argument function, use
.x
and.y
For more arguments, use
..1
,..2
,..3
etc
This syntax allows you to create very compact anonymous functions.
If character vector, numeric vector, or list, it is converted to an extractor function. Character vectors index by name and numeric vectors index by position; use a list to index by position and name at different levels. If a component is not present, the value of
.default
will be returned.- ...
Additional arguments passed on to the mapped function.
- .options
The
future
specific options to use with the workers. This must be the result from a call tofurrr_options()
.- .env_globals
The environment to look for globals required by
.x
and...
. Globals required by.f
are looked up in the function environment of.f
.- .progress
A single logical. Should a progress bar be displayed? Only works with multisession, multicore, and multiprocess futures. Note that if a multicore/multisession future falls back to sequential, then a progress bar will not be displayed.
Warning: The
.progress
argument will be deprecated and removed in a future version of furrr in favor of using the more robust progressr package.- .id
Either a string or
NULL
. If a string, the output will contain a variable with that name, storing either the name (if.x
is named) or the index (if.x
is unnamed) of the input. IfNULL
, the default, no variable will be created.Only applies to
_dfr
variant.